Introduction
In modern mobile application development, visual appeal and user experience play crucial roles in an app's success. UI/UX designers constantly strive to create engaging and aesthetically pleasing interfaces. One of the most effective ways to enhance visual appeal is through gradient backgrounds, which have become increasingly popular in mobile app design. In this comprehensive guide, we'll explore how to implement beautiful linear gradient backgrounds in your React Native applications.
Initialization
To begin, let's create a new React Native project using Expo CLI:
npx create-expo-app@latest --template blank-typescript react-native-lineargradient
Navigate to your project directory:
cd react-native-lineargradient
Installing Dependencies
We'll use Expo's linear gradient library. Install it with:
npx expo install expo-linear-gradient
Starting the Emulator:
Start your development server with:
npx expo start
The emulator is up and running!
The LinearGradient
component is straightforward to use. It requires color arrays and optional start/end points to control the gradient direction.
Using LinearGradient
is very easy, all you need are color arrays and start/end points. While start/end points have default settings, you can modify the color direction if desired.
Crafting a Simple Instagram Sign In Button with Linear Gradient Background
import React from 'react';
import { Text, View, TouchableOpacity, StyleSheet } from 'react-native';
import { LinearGradient } from 'expo-linear-gradient';
export default function App() {
return (
<View style={styles.container}>
<TouchableOpacity activeOpacity={0.8}>
<LinearGradient
colors={['#feda75', '#fa7e1e', '#d62976', '#962fbf', '#4f5bd5']}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 1 }}
style={styles.button}>
<Text style={styles.buttonText}>Sign in with Instagram</Text>
</LinearGradient>
</TouchableOpacity>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
padding: 18,
},
button: {
paddingVertical: 14,
paddingHorizontal: 30,
borderRadius: 30,
alignItems: 'center',
},
buttonText: {
color: '#fff',
fontWeight: '600',
fontSize: 16,
},
});
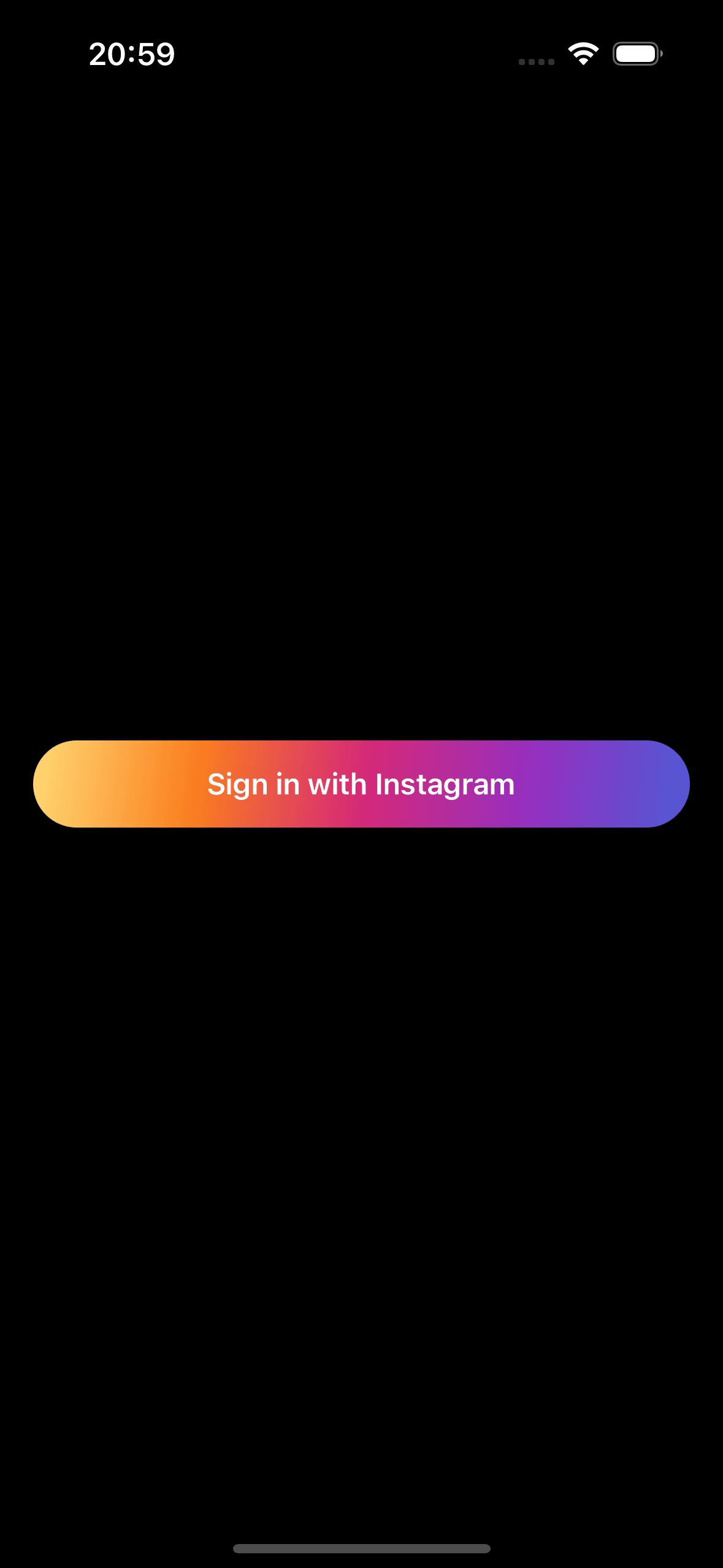
Customizing Gradient Direction
You can control the gradient flow by adjusting the start and end properties. Here's an example:
import { View } from 'react-native';
import { LinearGradient } from 'expo-linear-gradient';
export default function App() {
return (
<View style={{ flex: 1, justifyContent: 'center', padding: 18 }}>
<LinearGradient
colors={['#405de6', '#5851db', '#833ab4', '#c13584', '#e1306c', '#fd1d1d']}
start={[0, 0]}
end={[1, 1]}
style={{ justifyContent: 'center', alignItems: 'center', height: 250, borderRadius: 10 }}>
</LinearGradient>
</View>
);
}
Imagine the background image as a grid with X and Y axes. The top-left point corresponds to 0 (start prop), and the bottom-right point corresponds to 1 (end prop).
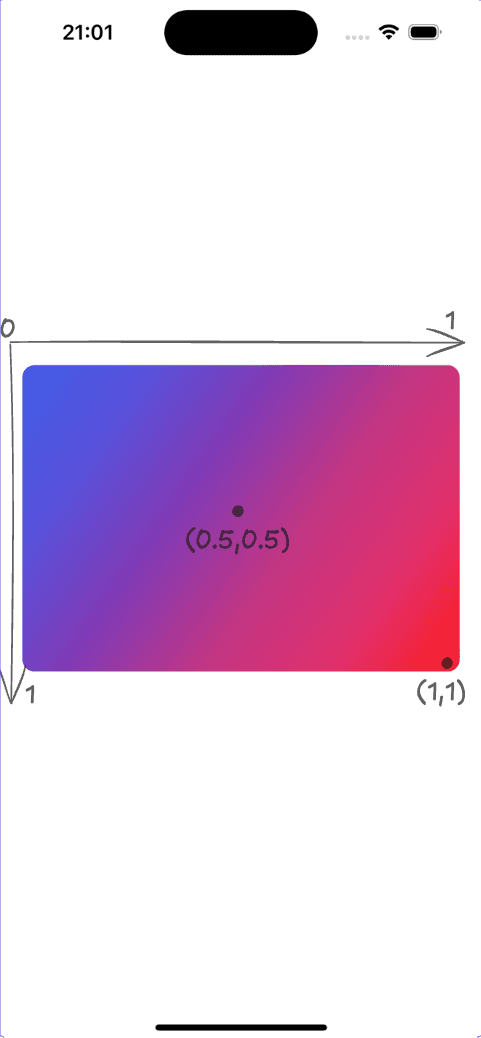
Exploring Beautiful Gradient Combinations
For inspiration and ready-to-use gradient combinations, visit uiGradients.
Here's a collection of stunning gradient combinations you can use in your projects:
import React from 'react';
import {
Text,
ScrollView,
TouchableOpacity,
StyleSheet,
} from 'react-native';
import { LinearGradient } from 'expo-linear-gradient';
const gradients = [
{ name: 'Sunset', colors: ['#ff7e5f', '#feb47b'] },
{ name: 'Instagram', colors: ['#feda75', '#fa7e1e', '#d62976', '#962fbf', '#4f5bd5'] },
{ name: 'Ocean Blue', colors: ['#2193b0', '#6dd5ed'] },
{ name: 'Purple Love', colors: ['#cc2b5e', '#753a88'] },
{ name: 'Aqua Marine', colors: ['#1A2980', '#26D0CE'] },
{ name: 'Bloody Mary', colors: ['#FF512F', '#DD2476'] },
{ name: 'Mojito', colors: ['#1D976C', '#93F9B9'] },
{ name: 'Flare', colors: ['#f12711', '#f5af19'] },
{ name: 'Green Beach', colors: ['#02AAB0', '#00CDAC'] },
{ name: 'Grapefruit Sunset', colors: ['#e96443', '#904e95'] },
{ name: 'Shifter', colors: ['#bc4e9c', '#f80759'] },
{ name: 'Emerald Water', colors: ['#348F50', '#56B4D3'] },
{ name: 'Cool Blues', colors: ['#2193b0', '#6dd5ed'] },
{ name: 'MegaTron', colors: ['#C6FFDD', '#FBD786', '#f7797d'] },
{ name: 'Pink Paradise', colors: ['#e1eec3', '#f05053'] },
{ name: 'Cherry', colors: ['#EB3349', '#F45C43'] },
{ name: 'Sky', colors: ['#76b852', '#8DC26F'] },
{ name: 'Purple Bliss', colors: ['#360033', '#0b8793'] },
{ name: 'Steel Gray', colors: ['#485563', '#29323c'] },
{ name: 'Peach', colors: ['#ED4264', '#FFEDBC'] },
];
export default function App() {
return (
<ScrollView contentContainerStyle={styles.container}>
{gradients.map((gradient, index) => (
<TouchableOpacity key={index} activeOpacity={0.8} style={styles.buttonWrapper}>
<LinearGradient
colors={gradient.colors}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 1 }}
style={styles.button}>
<Text style={styles.buttonText}>{gradient.name}</Text>
</LinearGradient>
</TouchableOpacity>
))}
</ScrollView>
);
}
const styles = StyleSheet.create({
container: {
padding: 16,
paddingTop: 50,
alignItems: 'center',
},
buttonWrapper: {
width: '100%',
marginBottom: 14,
},
button: {
borderRadius: 16,
paddingVertical: 32,
paddingHorizontal: 24,
alignItems: 'center',
justifyContent: 'center',
elevation: 2,
shadowColor: '#000',
shadowOpacity: 0.15,
shadowRadius: 4,
shadowOffset: { width: 0, height: 2 },
},
buttonText: {
color: '#fff',
fontSize: 16,
fontWeight: '600',
},
});
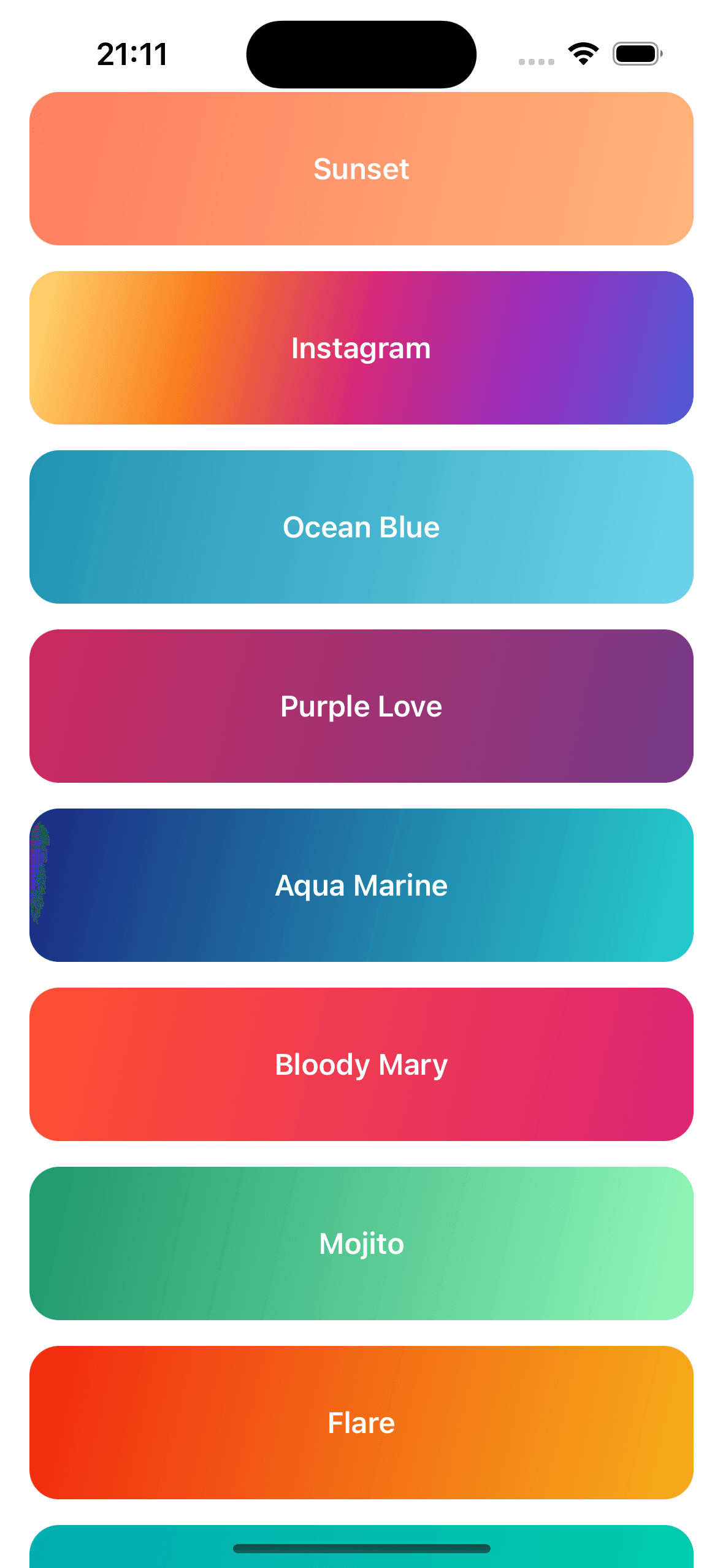
Source Code
Required Dependencies
"dependencies": {
"expo": "~53.0.9",
"expo-status-bar": "~2.2.3",
"react": "19.0.0",
"react-native": "0.79.2",
"expo-linear-gradient": "~14.1.4"
},
"devDependencies": {
"@babel/core": "^7.25.2",
"@types/react": "~19.0.10",
"typescript": "~5.8.3"
},
Conclusion
Implementing linear gradient backgrounds in React Native applications is a powerful way to enhance your app's visual appeal and user experience. With the expo-linear-gradient
component, you can easily create smooth, professional-looking color transitions that make your app stand out. Whether you're building a social media app, e-commerce platform, or any other mobile application, gradient backgrounds can significantly improve your UI design. Start experimenting with different color combinations and gradient directions to create unique and engaging user interfaces for your React Native applications.